Passing and Returning Object from Function in C++ Programming
- In C++ programming, objects can be passed to function in similar way as variables and structures.
Example to Pass Object to Function
C++ program to add two complex numbers by passing objects to function.
int imag;
public:
Complex(): real(0), imag(0) { }
void Read()
{
cout<<"Enter real and imaginary number respectively:"<<endl;
cin>>real>>imag;
}
void Add(Complex comp1,Complex comp2)
{
real=comp1.real+comp2.real;
/* Here, real represents the real data of object c3 because this function is called using code c3.add(c1,c2); */
imag=comp1.imag+comp2.imag;
/* Here, imag represents the imag data of object c3 because this function is called using code c3.add(c1,c2); */
}
void Display()
{
cout<<"Sum="<<real<<"+"<<imag<<"i";
}
};int main()
{
Complex c1,c2,c3;
c1.Read();
c2.Read();
c3.Add(c1,c2);
c3.Display();
return 0;
}
Expected Output:-
Enter real and imaginary number respectively:
12
3
Enter real and imaginary number respectively:
2
6
Sum=14+9i
Returning Object from Function
- The syntax and procedure to return object is similar to that of returning structure from function.
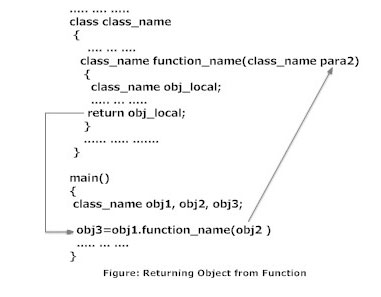
Example to Return Object from Function
- This program is the modification of above program displays exactly same output as above.
- But, in this program, object is return from function to perform this task.
#include <iostream>
using namespace std;
class Complex
{
private:
int real;
int imag;
public:
Complex(): real(0), imag(0) { }
void Read()
{
cout<<"Enter real and imaginary number respectively:"<<endl;
cin>>real>>imag;
}
Complex Add(Complex comp2)
{
Complex temp;
temp.real=real+comp2.real;
/* Here, real represents the real data of object c1 because this function is called using code c1.Add(c2) */
temp.imag=imag+comp2.imag;
/* Here, imag represents the imag data of object c1 because this function is called using code c1.Add(c2) */
return temp;
}
void Display()
{
cout<<"Sum="<<real<<"+"<<imag<<"i";
}
};
int main()
{
Complex c1,c2,c3;
c1.Read();
c2.Read();
c3=c1.Add(c2);
c3.Display();
return 0;
}
Tags:
c++ programming
manjeet singh kuthar
mskuthar
Object and Function in c++
www.mskuthar.blogspot.com