Tricky c programs question for interview and answers with explanation. These questions are for experienced persons.
C advanced interview questions and answers
(1) What will be output if you will compile and execute the following c code?
struct marks{
int p:3;
int c:3;
int m:2;
};
void main(){
struct marks s={2,-6,5};
printf("%d %d %d",s.p,s.c,s.m);
}
(a) 2 -6 5
(b) 2 -6 1
(c) 2 2 1
(d) Compiler error
(e) None of these
Answer: (c)
Explanation:
Binary value of 2: 00000010 (Select three two bit)
Binary value of 6: 00000110
Binary value of -6: 11111001+1=11111010
(Select last three bit)
Binary value of 5: 00000101 (Select last two bit)
Complete memory representation:
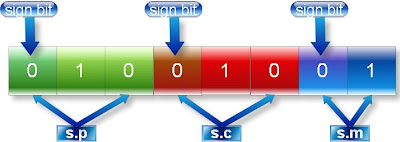
(2) What will be output if you will compile and execute the following c code?
void main(){
int huge*p=(int huge*)0XC0563331;
int huge*q=(int huge*)0xC2551341;
*p=200;
printf("%d",*q);
}
(a)0
(b)Garbage value
(c)null
(d) 200
(e)Compiler error
Answer: (d)
Explanation:
Physical address of huge pointer p
Huge address: 0XC0563331
Offset address: 0x3331
Segment address: 0XC056
Physical address= Segment address * 0X10 + Offset address
=0XC056 * 0X10 +0X3331
=0XC0560 + 0X3331
=0XC3891
Physical address of huge pointer q
Huge address: 0XC2551341
Offset address: 0x1341
Segment address: 0XC255
Physical address= Segment address * 0X10 + Offset address
=0XC255 * 0X10 +0X1341
=0XC2550 + 0X1341
=0XC3891
Since both huge pointers p and q are pointing same physical address so content of q will also same as content of q.
(3) Write c program which display mouse pointer and position of pointer.(In x coordinate, y coordinate)?
Answer:
#include”dos.h”
#include”stdio.h”
void main()
{
union REGS i,o;
int x,y,k;
//show mouse pointer
i.x.ax=1;
int86(0x33,&i,&o);
while(!kbhit()) //its value will false when we hit key in the key board
{
i.x.ax=3; //get mouse position
x=o.x.cx;
y=o.x.dx;
clrscr();
printf("(%d , %d)",x,y);
delay(250);
int86(0x33,&i,&o);
}
getch();
}
(4) Write a c program to create dos command: dir.
Answer:
Step 1: Write following code.
#include “stdio.h”
#include “dos.h”
void main(int count,char *argv[]){
struct find_t q ;
int a;
if(count==1)
argv[1]="*.*";
a = _dos_findfirst(argv[1],1,&q);
if(a==0){
while (!a){
printf(" %s\n", q.name);
a = _dos_findnext(&q);
}
}
else{
printf("File not found");
}
}
Step 2: Save the as list.c (You can give any name)
Step 3: Compile and execute the file.
Step 4: Write click on My computer of Window XP operating system and select properties.
Step 5: Select Advanced -> Environment Variables
Step 6: You will find following window:
Click on new button (Button inside the red box)

Step 7: Write following:
Variable name: path
Variable value: c:\tc\bin\list.c (Path where you have saved)
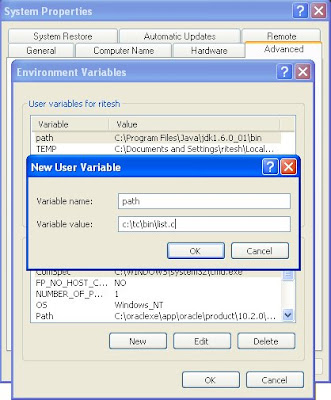
Step 8: Open command prompt and write list and press enter.
Command line argument tutorial.
(6) What will be output if you will compile and execute the following c code?
void main(){
int i=10;
static int x=i;
if(x==i)
printf("Equal");
else if(x>i)
printf("Greater than");
else
printf("Less than");
}
(a) Equal
(b) Greater than
(c) Less than
(d) Compiler error
(e) None of above
Answer: (d)
Explanation:
static variables are load time entity while auto variables are run time entity. We can not initialize any load time variable by the run time variable.
In this example i is run time variable while x is load time variable.